Resources are the additional files and static content that your code uses, such as bitmaps, layout definitions, user interface strings, animation instructions, and more.
You should always externalise app resources such as images and strings from your code, so that you can maintain them independently.
Once you externalise your app resources, you can access them using resource IDs that are generated in your project’s R class.
You should place each type of resources in a specific subdirectory of your project’s res/ directory.
animator: XML files that define property animations.
anim: XML files that define tween animations. (Property animations can also be saved in this directory, but the animator / directory is preferred for property animations to distinguish between the two types)
Color: XML files that define a state list of colors
drawable: Bitmap files (.png, 9.png, .jpg, .gif) or XML files that are compiled into the following drawable resource subtypes:
- Bitmap files
- Nine-Patches (re-sizable bitmaps)
- State lists
- Shapes
- Animation drawables
- Other drawables
mipmap: Drawable files for different launcher icon densities.
layout: XML files that define a user interface layout
menu: XML files that define app menu, such as an Option Menu, Context Menu, or Sub Menu.
raw: Arbitrary files to save in their raw form
values: XML files that contain simple values, such as strings, integers, and colors.
xml: Arbitrary XML files that can be read at runtime by calling Resources.getXML()
Font: Font files with extensions such as .ttf, .otf, or .ttc, or XML files that include a <font-family> element.
Colors
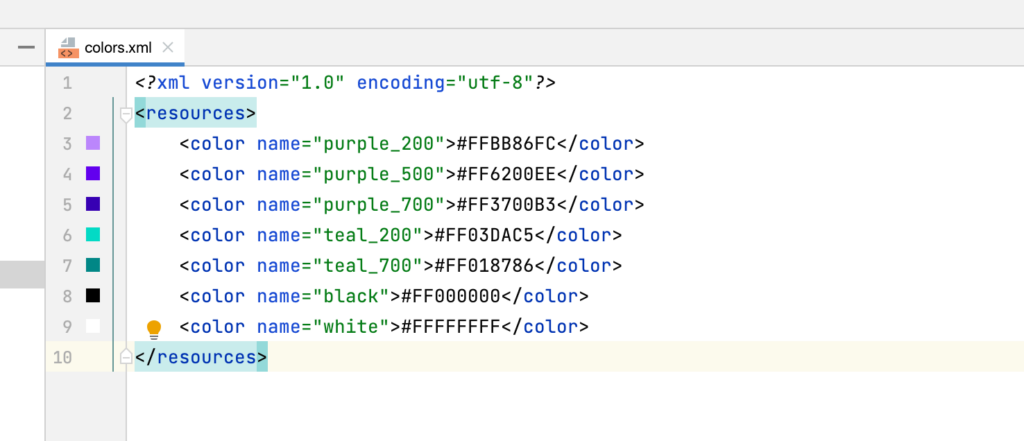
Getting Color from Code:
// Kotlin
var color: Int = resources.getColor(R.color.white)
// Java
Resources res = getResources();
int white_color = res = res.getColor(R.color.white)
Strings
String:

Array:
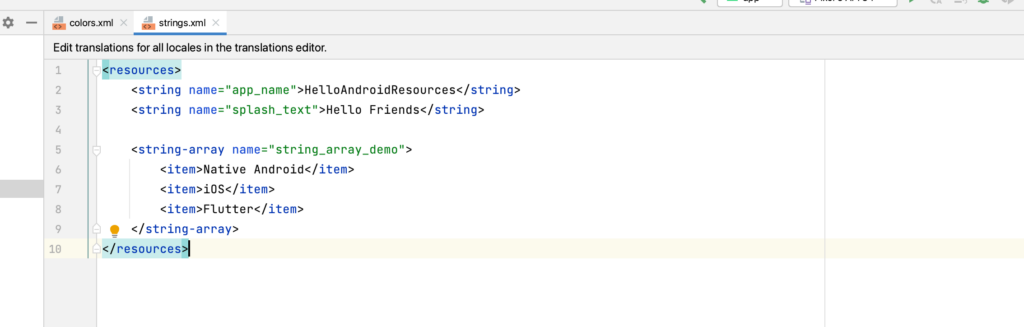
Menu
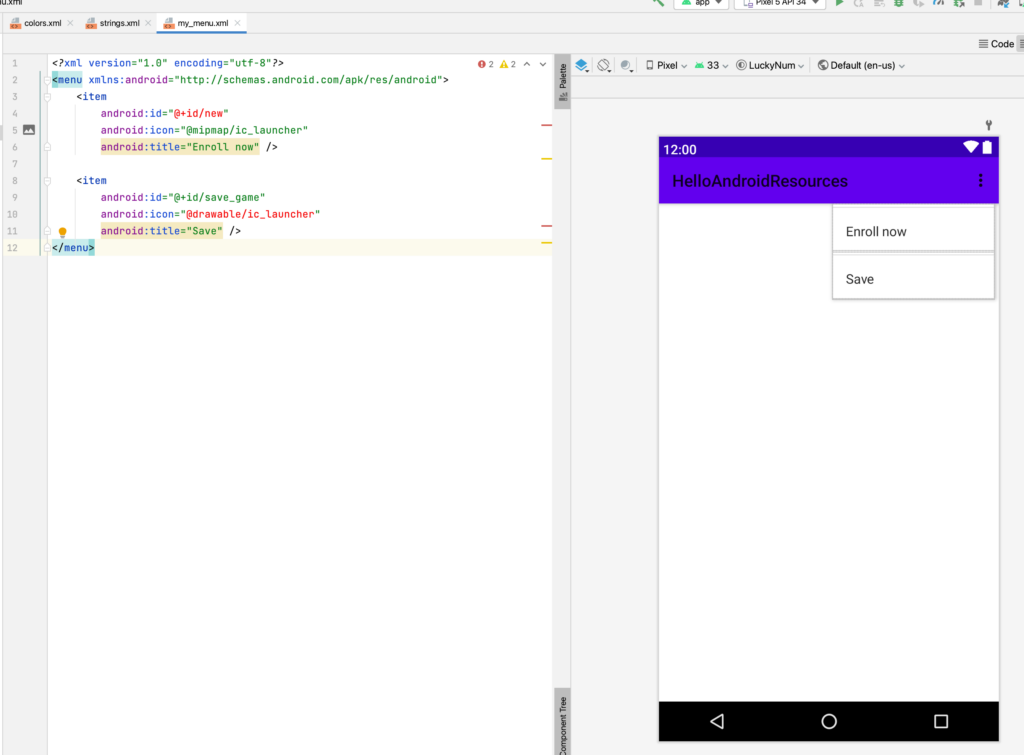
// Java
@Override
public boolean onCreateOptionsMenu(Menu menu) {
MenuInflater inflater = getMenuInflater();
inflater = inflater.inflate(R.menu.my_menu, menu);
return true;
}
// Kotlin
override fun onCreateOptionsMenu(menu: Menu) Boolean {
menuIdentifier.inflate(R.menu.my_menu, menu)
return true
}
Drawable
Gradient Background using drawable gradient xml
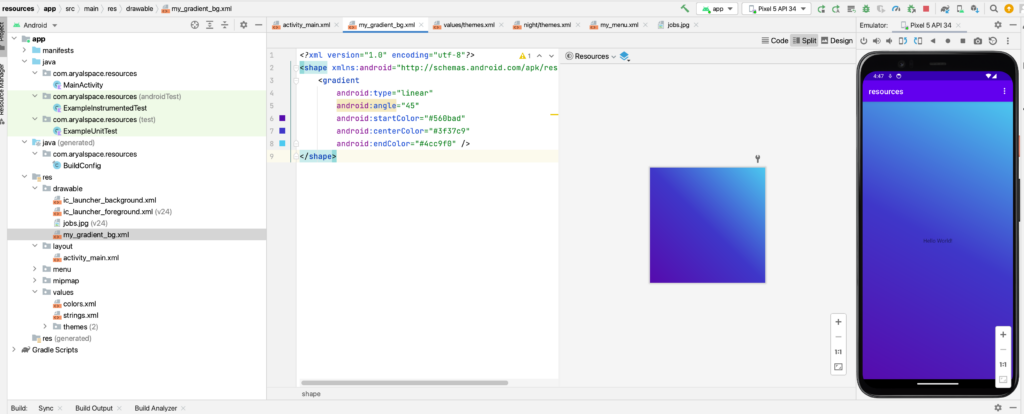
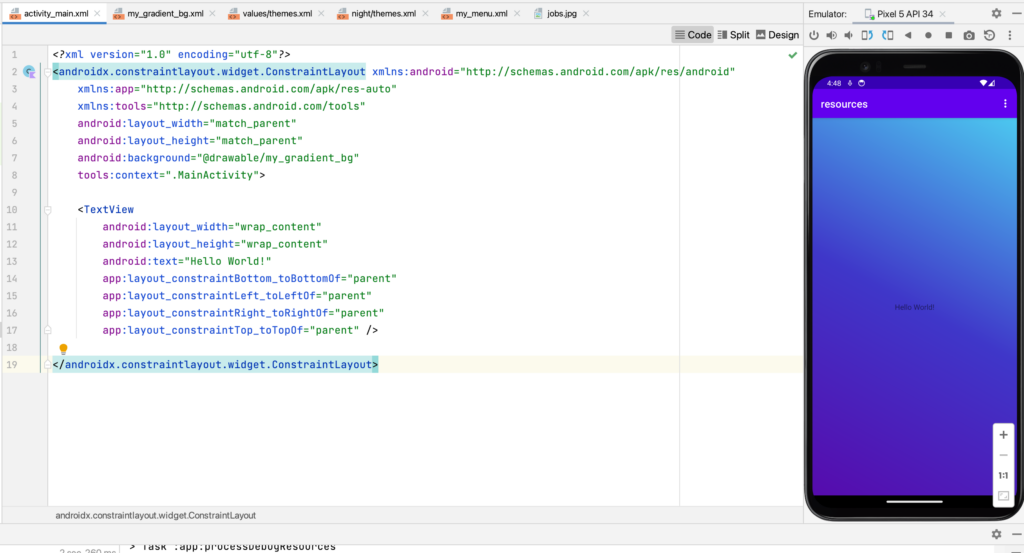
Custom Shapes
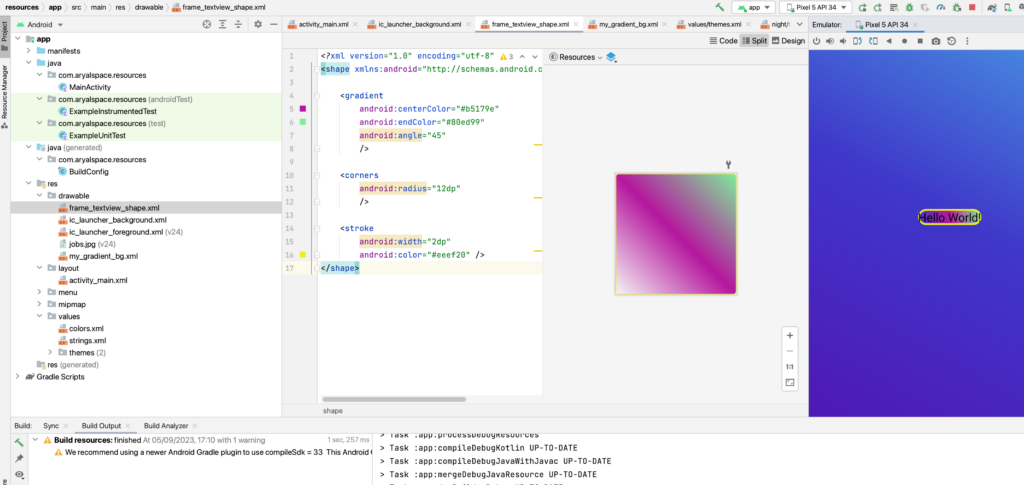
Fonts
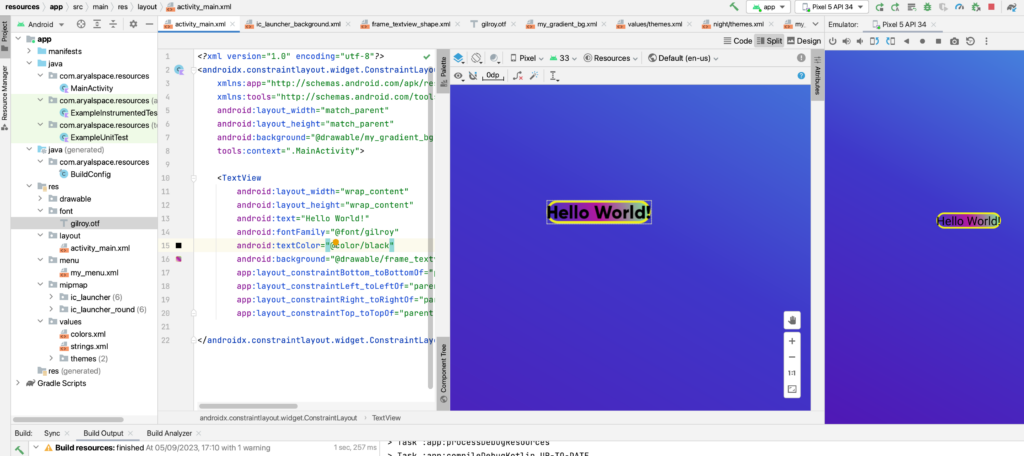